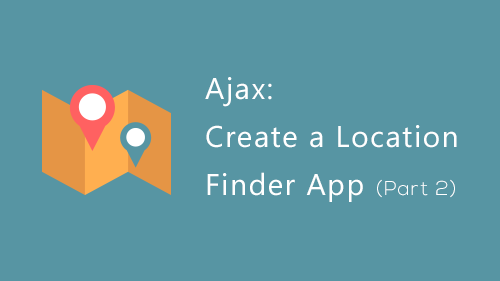
Enroll in the training to get access to this lesson along with hundreds of other lessons and complete courses.
Already enrolled? Login
Ajax: Create a Location Finder App using jQuery & Google Maps API Part 2
Continuing from our previous Create a Location Finder App using jQuery & Google Maps API part 1 lesson. We know that longitude and latitude parameters are hard coded and it's not picking up the actual location the user is at. So we are going to use the feature that HTML5 introduces called the Geo location.
There's an object in the modern browsers called navigator, not all browsers have it, only the latest browser do. With the help of navigator you can see if the Geo location feature is available on your browser.
I'm going to write a function and call it getLocation
and I'll do navigator.geolocation
to see if the Geo Location feature is available in the browser, if that condition is true, then I'm going to call a function inside that object called navigator.geolocation.getCurrentPosition
and this will have a function in it which is going to run when the location is found and call the function (loc)
and for demonstration purposes, I'll console.log
function getLocation(){
if(navigator.geolocation){
navigator.geolocation.getCurrentPosition(function(loc){
console.log(loc);
});
}
}
The getLocation function is going to run immediately when the button (anchor tag) is clicked in the webpage. Save it, refresh the screen
Save it, refresh the screen and now if you click on the anchor tag one more time and wait a little bit, just after couple of seconds the Geo Location will display like such.
The navigator at the top is using the HTML5 feature of Geo location from the browser. I have already enabled and allowed the browser for our web server URL to access my location.
Well that's a privacy concern. Go to the Chrome settings if you're using chrome like I'm doing and scroll down go to the advanced settings. Go to content settings, scroll down to location and click manage exceptions and you can cancel it, if you're doing it for the first time.
Now that I have disabled it and if I refresh the page again and click it one more time, you will see the pop up asking you to either allow or deny and if you allot it, your location is accessible but if you deny it, your location is not accessible.
And if I hit allow, it will wait a few seconds then the location information will be printed as shown below.
Inside coords, we have latitude and longitude. Now our job is to pick up the latitude and longitude information, combine it and separate with a comma then pass it to the Google APIs.
I'm going to create a variable and call it latlng
and concatenate with a comma. Also getCity
function is going to be called when the location information is found. I'm going to pass latlng function to the getCity
.
function getLocation(){
if(navigator.geolocation){
navigator.geolocation.getCurrentPosition(function(loc){
var latlng = loc.coords.latitude + ',' + loc.coords.longitude;
getCity(latlng);
});
}
}
When the getCity is run, it needs the latlngInput parameter
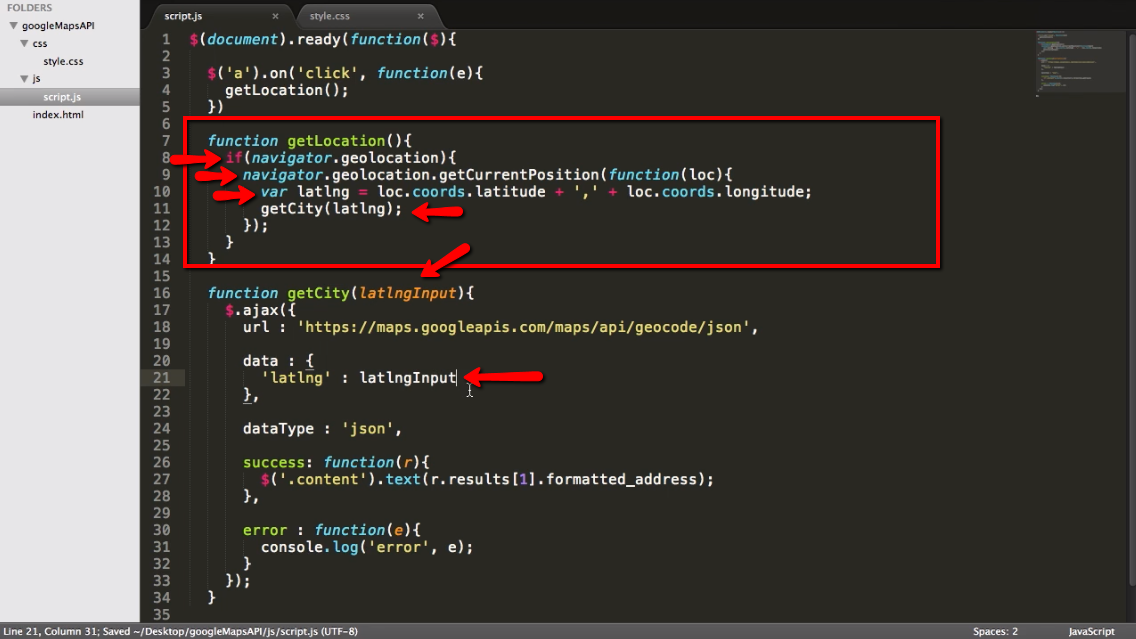
Save it, refresh the screen and click "What City Am I In?" and you will see the city gets printed on the screen and the Geo location is working fine. Also if you want to know how it works, you can go to Network tab and click on the file and the call is being made to the Google Maps APIs and if you go to the response tab, you will see all the information regarding the city, state, county and the zip code etc.
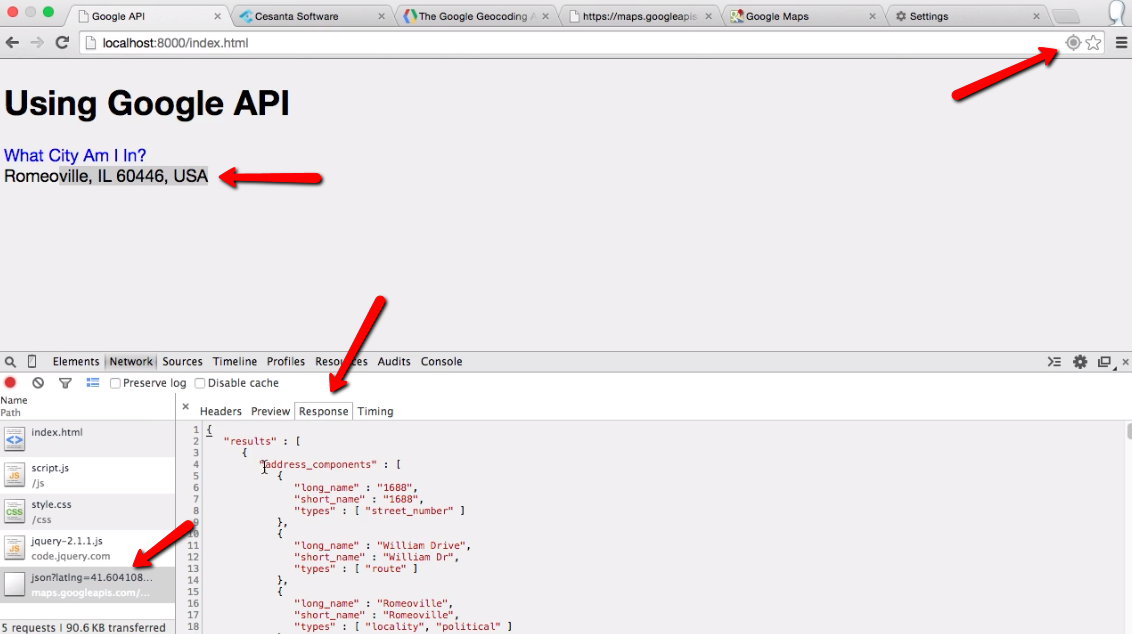
Get Started
Already have an account? Please Login