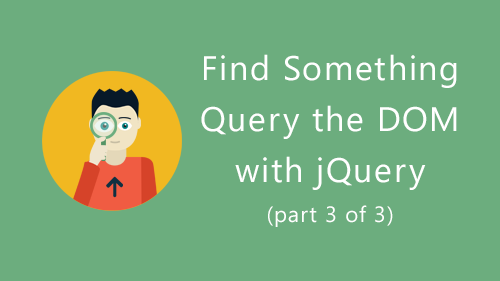
Enroll in the training to get access to this lesson along with hundreds of other lessons and complete courses.
Already enrolled? Login
What are Selectors in jQuery Part 3
In this lesson, we are going to look at another way to querying the DOM
Getting started
I have already created the document in HTML
I'm going to import jQuery in our head tag, go to the website and click on uncompressed version
It will lead you to another page, simply copy and paste the URL in the script tag
In this lesson, we are going to discuss the methods below to query the DOM
I'm going to test if our code is working
$(document).ready(function( ){
$('body').addclass('new');
});
Save it, refresh the screen and you see it added a class of new to the body which means our jQuery is working
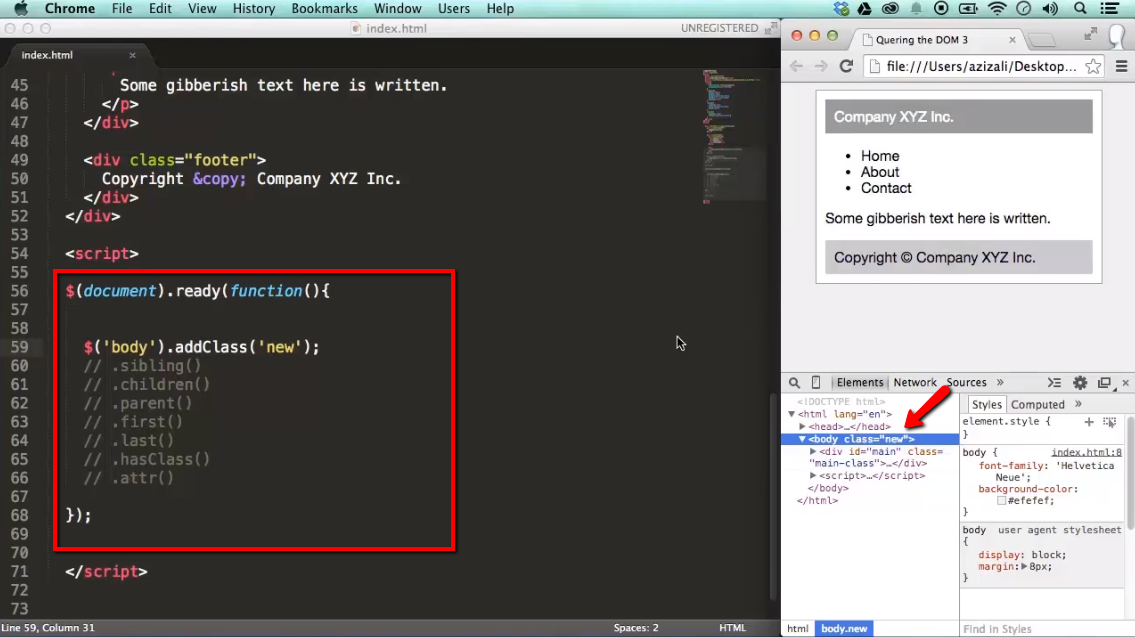
But before we proceed, I want to show you something cool. Open your chrome developer tools, go to the console and if I write some code like
$('body')
and hit enter you see it queried the DOM and brought me the body element from the DOM. Also you notice its surrounded by the brackets [ ] which means its an array.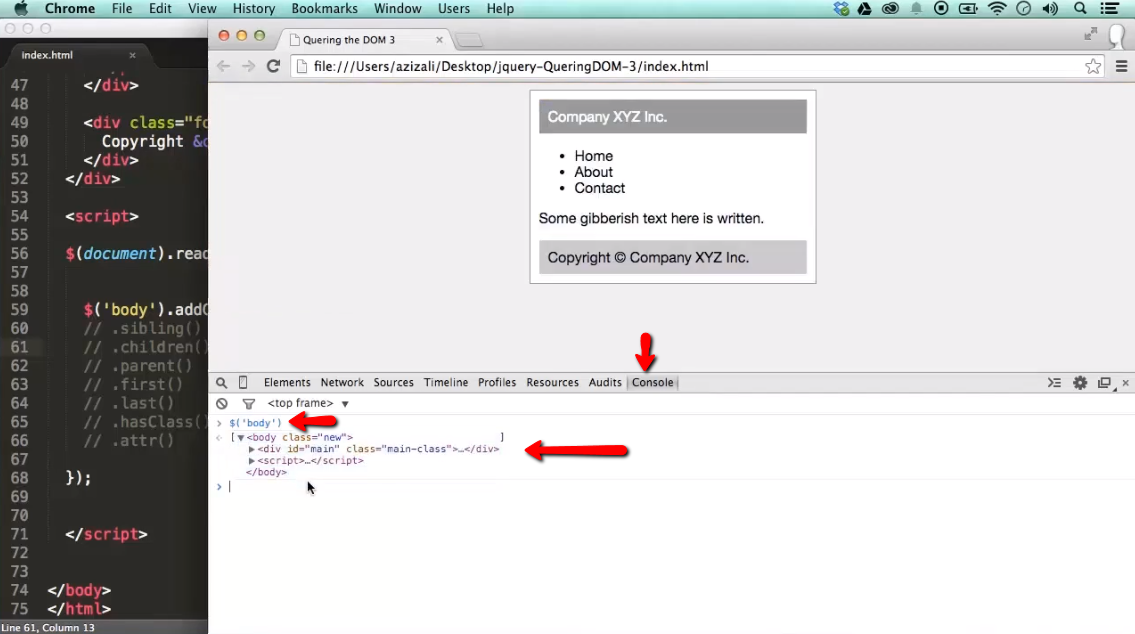
When we import jQuery in our project, it can also be accessed in the console. So for example if I write
$('p')
it's going to return the paragraph tags and same with the li tags as well $('li')
and the div tags as well $('div')
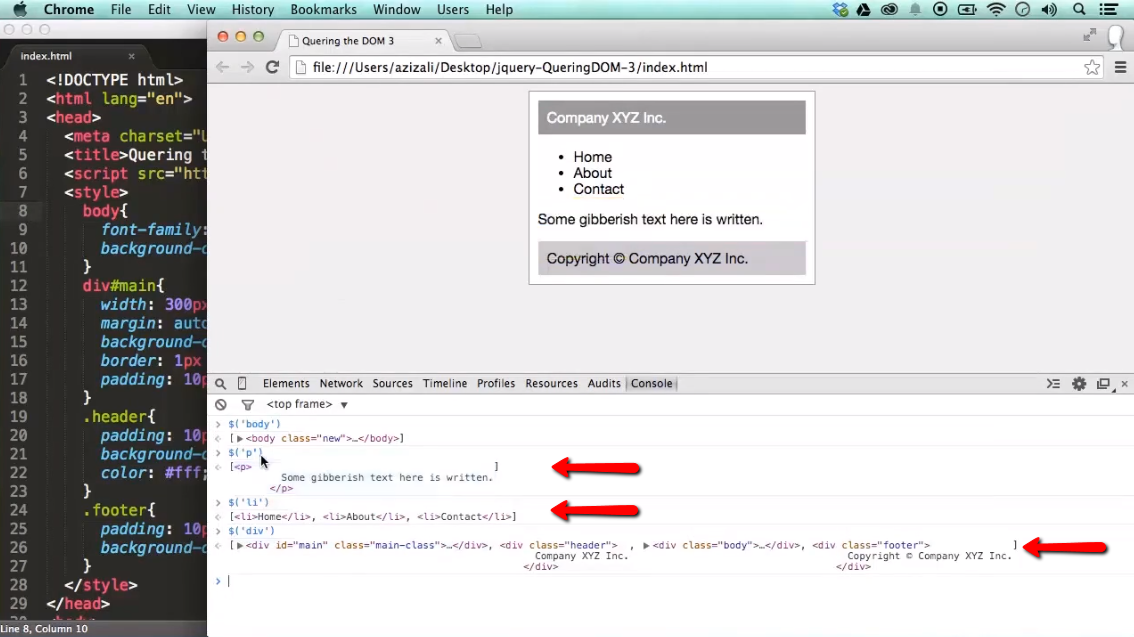
$('#main')
it means give me all the elements which have an ID of main, if I hit enter I only get 1 div because there's only one div in our HTML document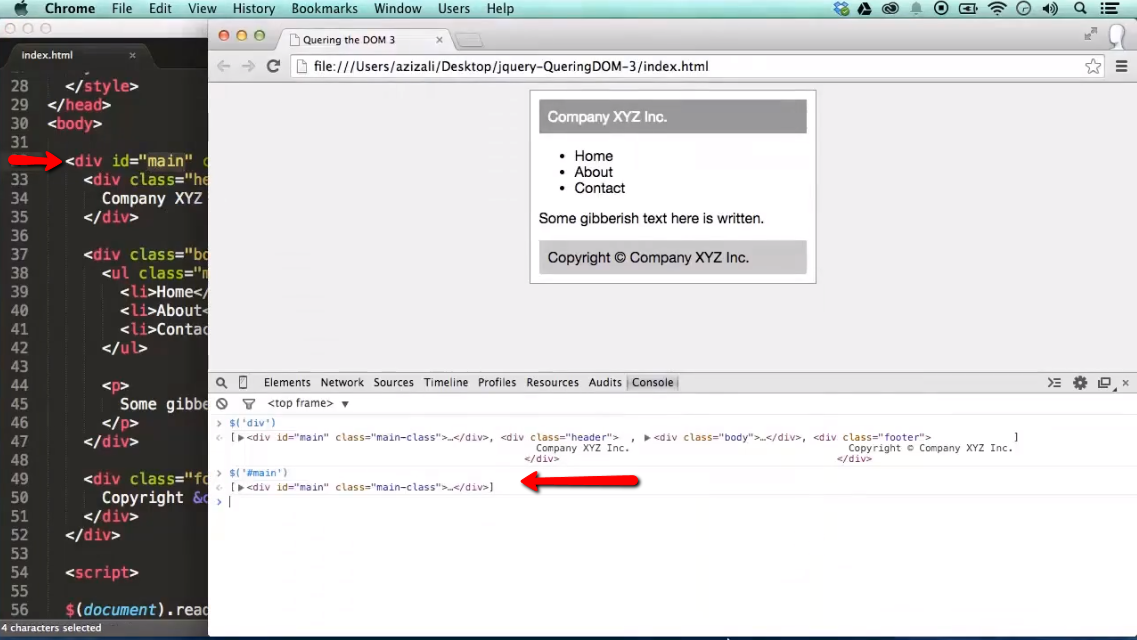
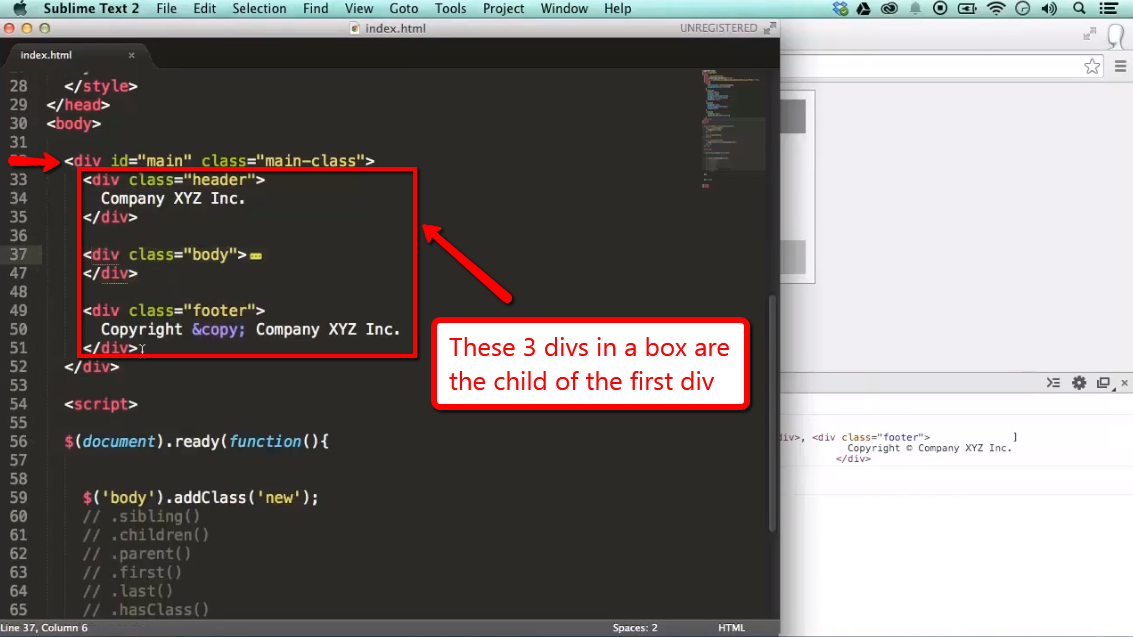
$('main').children( )
save it, refresh the screen you see the children of div are returned.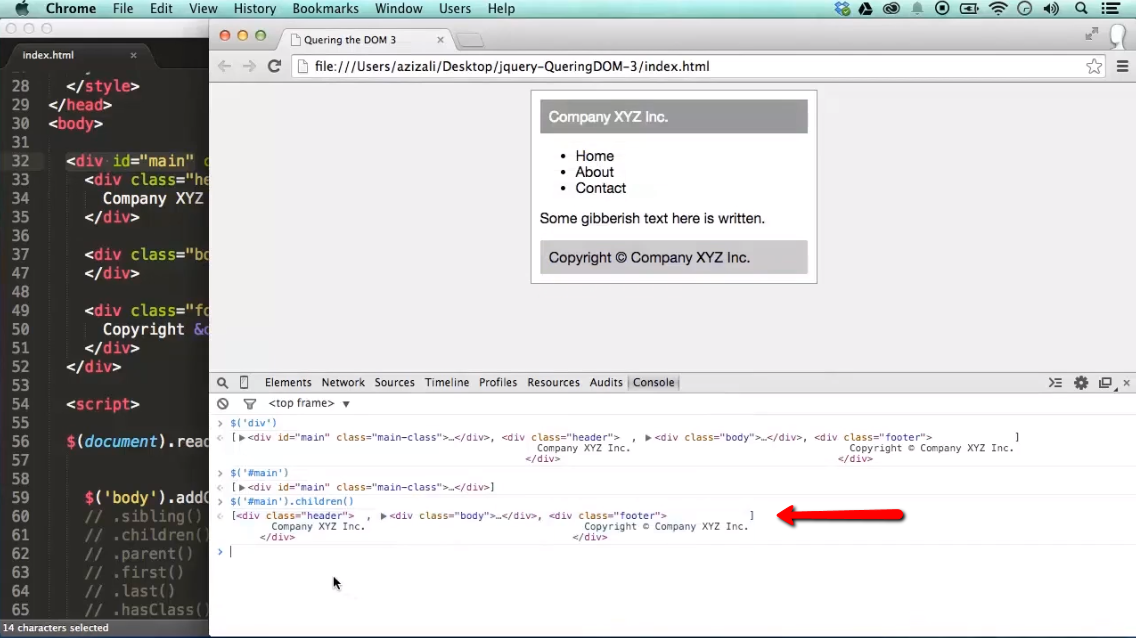
If you want the particular div as shown in a screenshot below
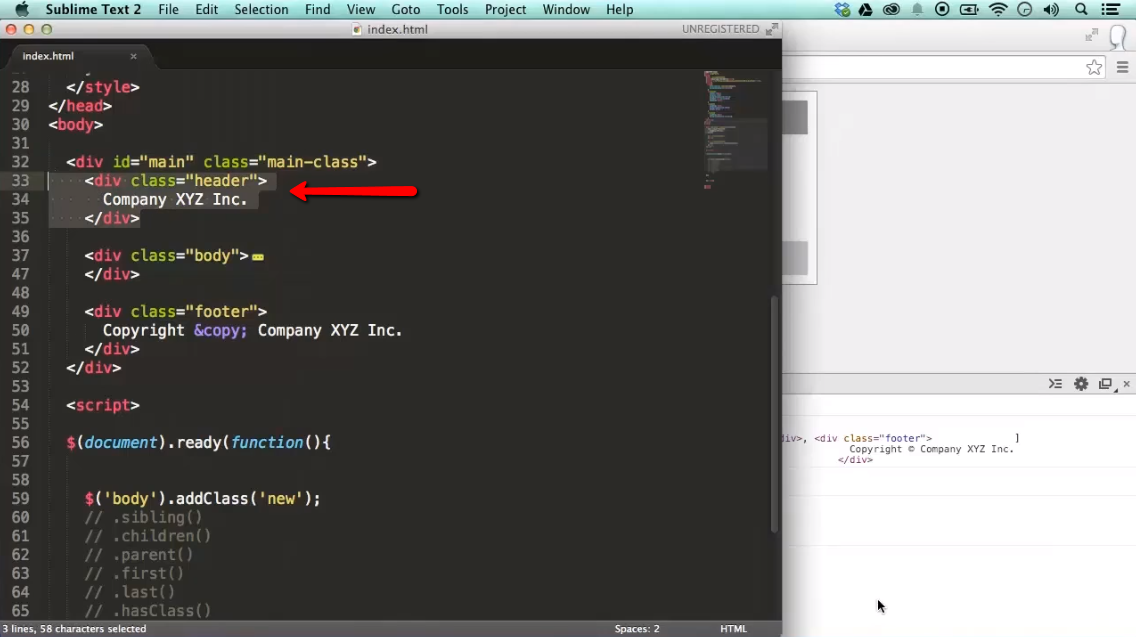
$('.header')
refresh the screen and you see it returned the first div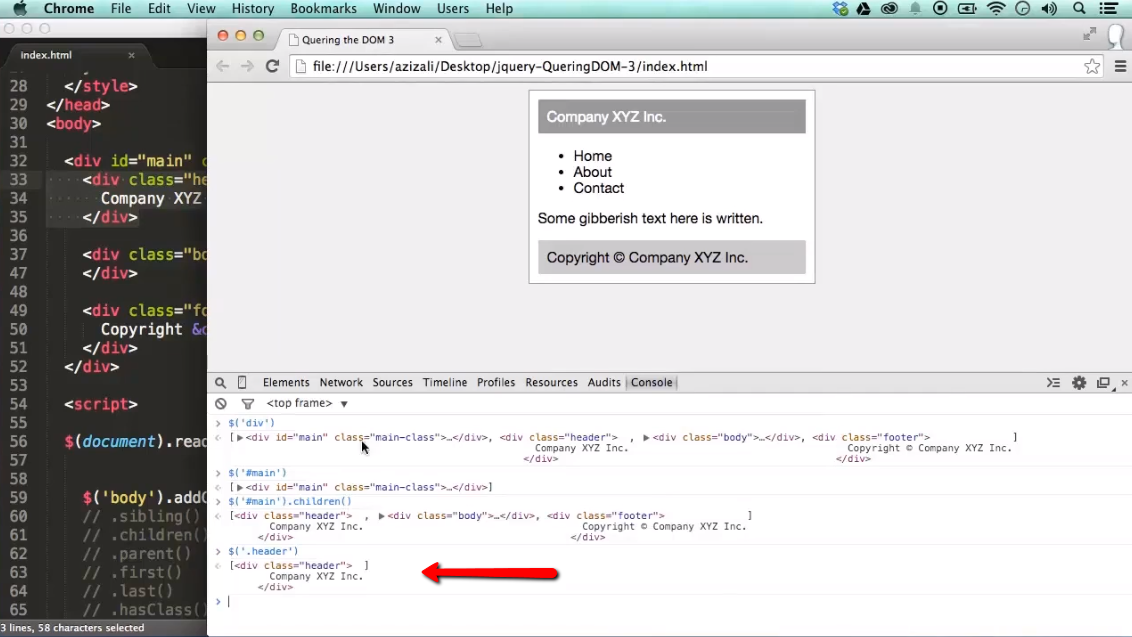
If you want the siblings of that particular div, I can simply write $('.header').siblings( )
hit enter and you see it returned the div siblings
So far we have looked at children and sibling methods of jQuery. We can also do that in our text editor as well.
.sibling( )
I can simply write
$('.header').sibling().css('color', 'red');
I can simply write
$('ul').children().css('color', 'green');
Save it, refresh the screen and you see all the siblings of the header have the color of red, and all the children of the ul tag have the color of green as shown below 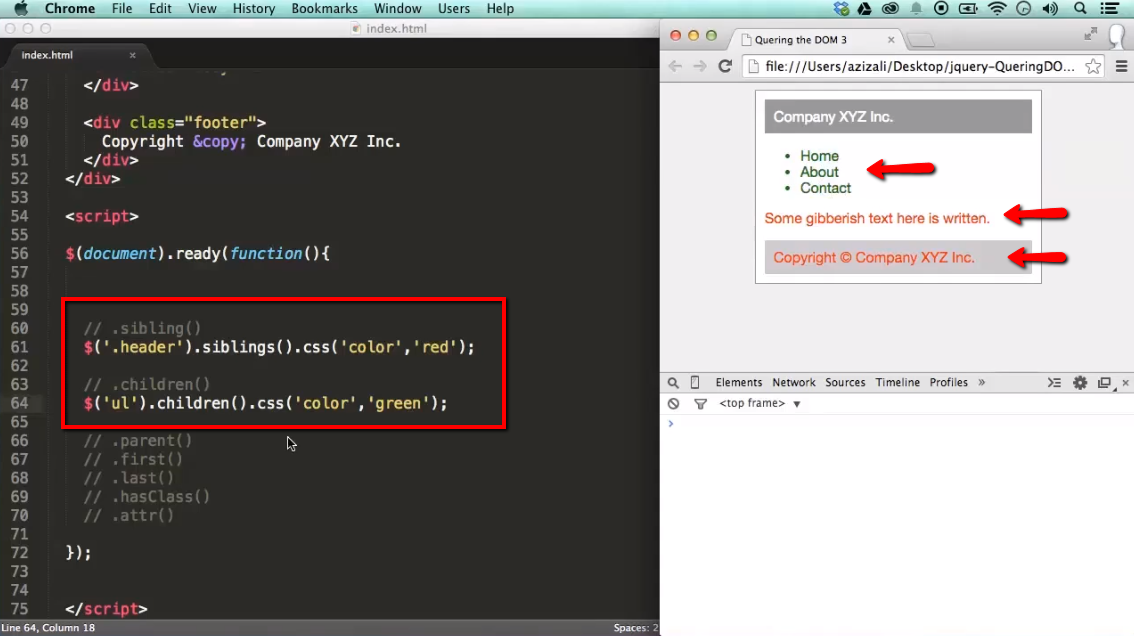
.parent( )
For the parent we can target the footer tag, and the div is the parent of that particular footer tag
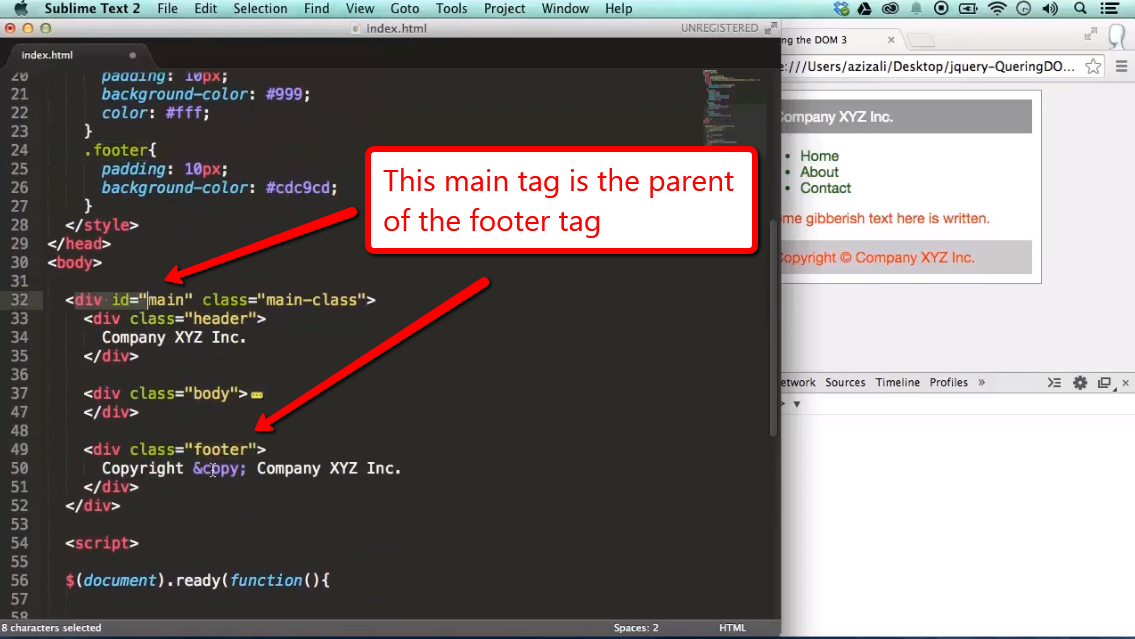
I can simply write
$('.footer').parent().css('background-color', 'blue');
Save it, refresh the screen and you see the background color is now changed to blue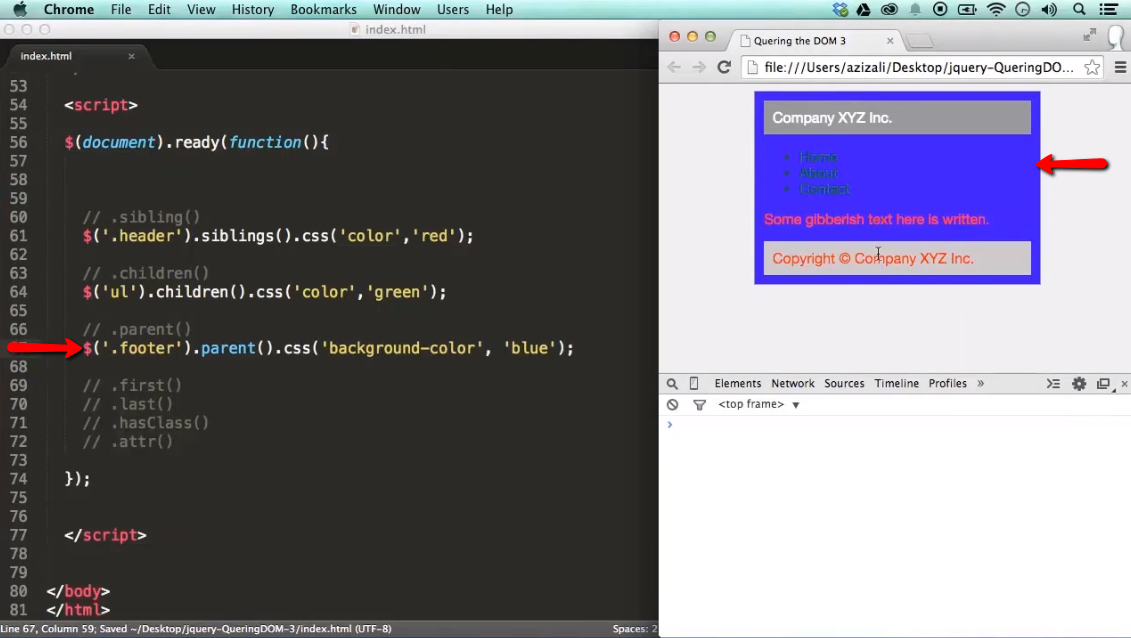
.first( )
If you want the first child and its color to brown you can simply write
$('ul').children().first().css('color', 'brown');
Save it, refresh the screen and you see the color is now changed to brown. you can also transform the text to uppercase or all lower case etc
you can also indent your code so it looks more readable like such
$('ul')
.children()
.first()
.css('color', 'brown');
You see the code is now more readable, also if you go to the console and write
$('ul')
you will get the ul tags and if you say you don't want the ul tag but you want its children as well so you can say $('ul').children()
and it will return its children. If you only want the first children you can simple write $('ul').children().first()
and it will return the fist children
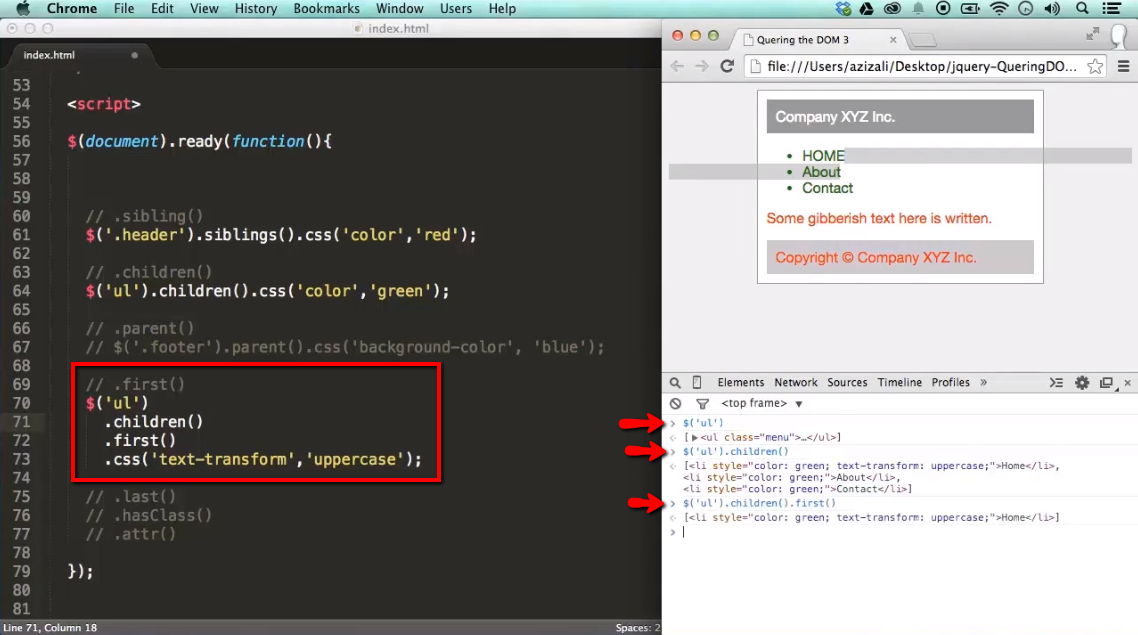
$('ul')
.children()
.last()
.css('text-transform', 'uppercase');
Save it, refresh the screen and you see the last one has the upper case now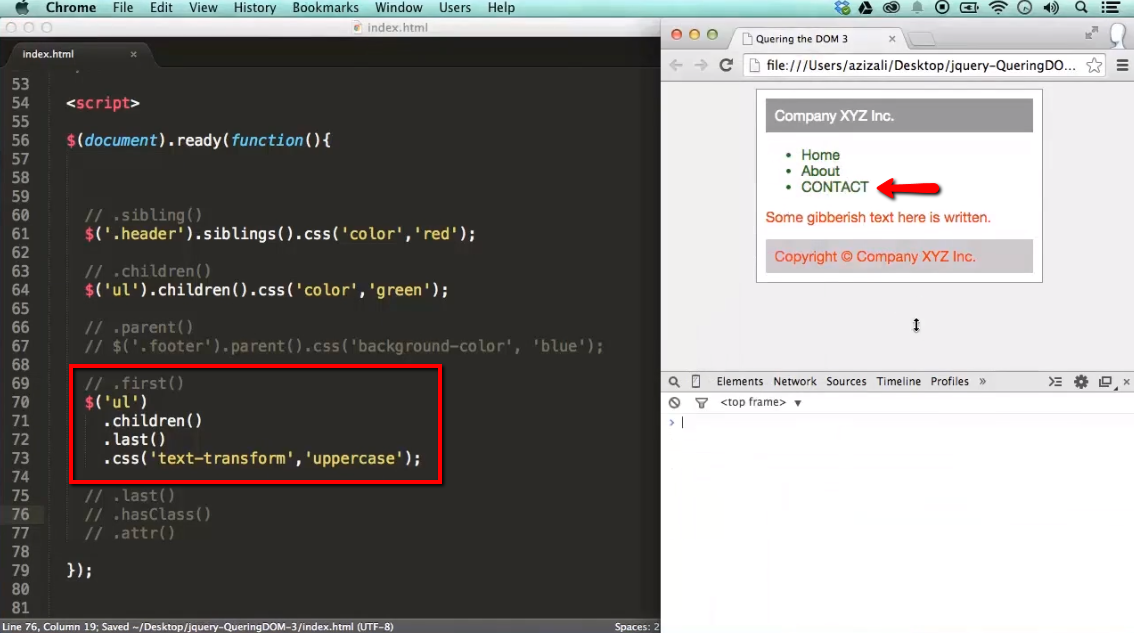
.hasClass( )
$('div')
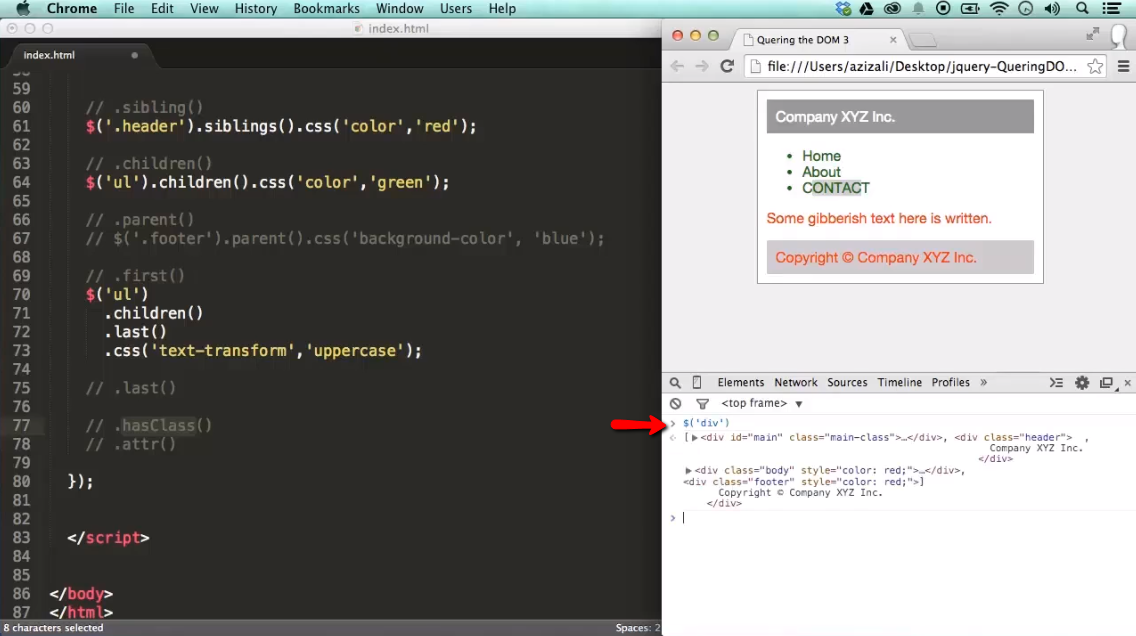
Then we are going to write div which has class of the header like such
$('div').hasClass('header')
save it, refresh the screen and you see it returned 'true' means it has a class of header. It's mostly a true or false statement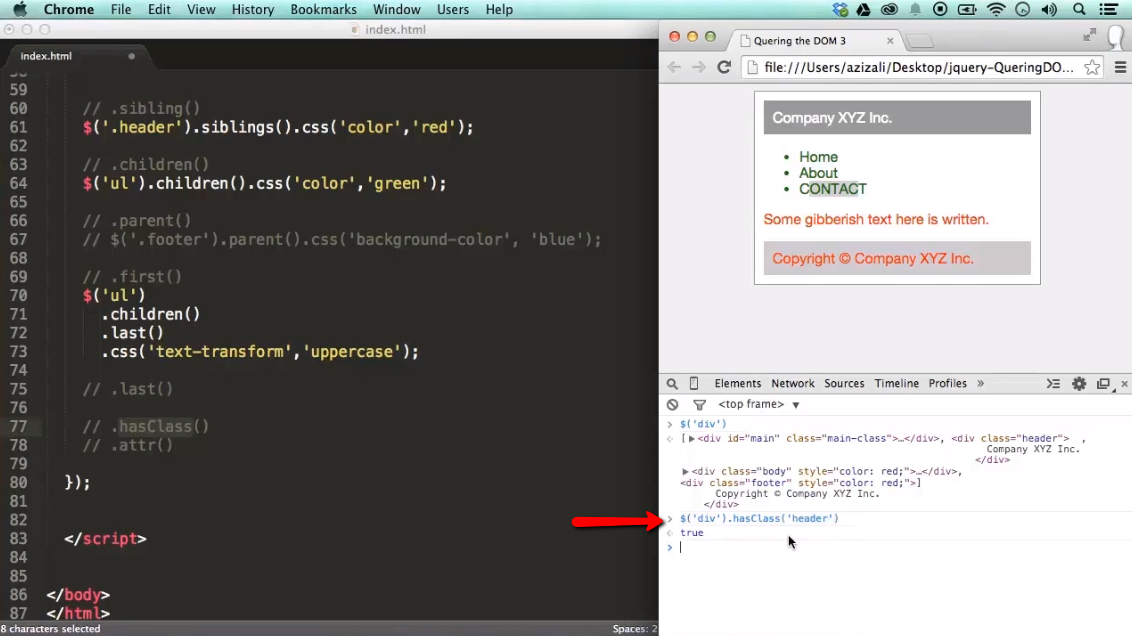
$('div').last( ).hasClass('header')
save it, refresh the screen and you see it will return 'false' because the last div has a class of footer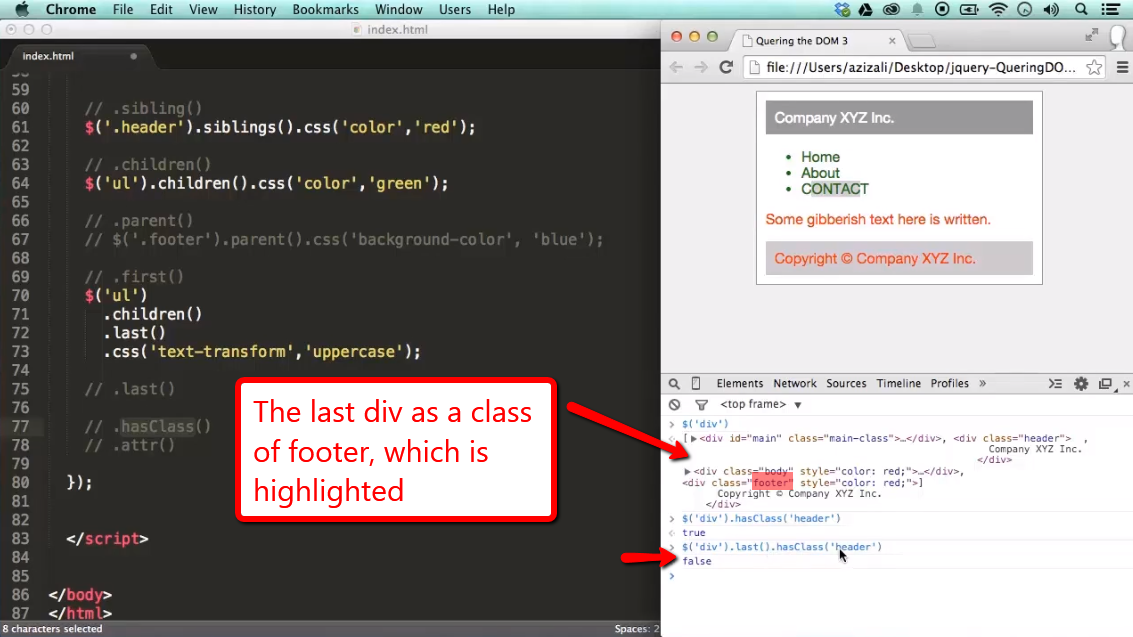
.attr( )
Now I'm going to look at the final method to query the DOM for today's lesson. If you have taken the Learn HTML & CSS in 14 Days course we have discussed what attributes are. Attributes provide additional information about an element. For example: The paragraphs are defined with the <p>
tag, in this example, the element has a title attribute. The value of the attribute is "iLoveCoding" for example
<p title="iLoveCoding">
This is some random text
</p>
So if I want to know attribute of the first id you can simply say $('div').first().attr('id')
hit enter and it will say the attribute for the id is main
If you want to know the class of the div, you can simply write $('div').first().attr('class')
save it, refresh it and hit enter you will see it returned the class of the div
So if I only write $('div')
it's going to return multiple results
If I want to find the attribute of the above results I can simply do $('div').attr('class')
and hit enter, it just gives the result of the first one
I hope you enjoyed this lesson, if you have any question, please leave your comments below. I'll talk to you in the next lesson. Goodbye :)
Get Started
Already have an account? Please Login